Throw Defect
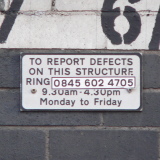
While I'm on the subject of exceptions, let me share another exception idiom I've used a lot on recent projects... the Defect exception.
The Java compiler is very strict. It will complain about missing code paths that you, the programmer, know should never be followed at runtime. This is especially common when using APIs that throw checked exceptions to process data that will always be correct unless the programmer has screwed up..
For example, loading and parsing template from a resource that is compiled into the application should never fail, but throws a checked IOException.
Template template; try { template = new Template(getClass().getResource("data-that-is-compiled-into-the-app.xml")); } catch (IOException e) { // should never happen } ...
If an IOException is caught, something is seriously wrong with the application itself, because of something the programmer has done. The application has been built incorrectly, perhaps, or the template is syntactically incorrect. If the application continues running it will fail with confusing errors later on. The error will be difficult to diagnose.
So, the catch block must throw another exception. It can't throw a checked exception. Because it has caught a programming error, it should throw some kind of RuntimeException. RuntimeException itself is a bit vague, and there isn't a subclass of RuntimeException that really fits the bill for this situation. Therefore, I like to define a new exception type to report programmer errors. I first called it StupidProgrammerException, but now, as suggested by Romilly, I call it by the less confrontational name of Defect:
public class Defect extends RuntimeException { public Defect(String message) { super(message); } public Defect(String message, Throwable cause) { super(message, cause); } }
When the compiler asks me to write code paths that should never happen, I throw a Defect. For example:
Template template; try { template = new Template(getClass().getResource("data-that-is-compiled-into-the-app.xml")); } catch (IOException e) { throw new Defect("could not load template", e); } ...